This article focus on generating image on fly in asp.net. This is very basic functionality which you find on almost all the website which require registration of user. Example: Yahoo Registration require to type you secret string before you can successfully login, this feature has no. of reasons and i am not going into detail of why we want rather i am explaining here how we can. Look for Screenshot for better idea
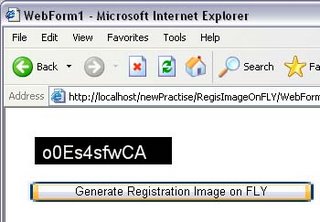
For demo purpose i am generating image on button click. But generally you require to generate image on page load event of registration form.
Ok back on coding.
Step1: Create a new web application
Step2: Open Code Behind Form of web application you have created.
Step3: Add Namespace for drawing Image.
using System.Drawing;
using System.Drawing.Imaging;
Step4: Write Code for Generating Random Registration String (Here: Generating Random string of 10 in length).
// Generate a random password with the specified length. The password will only
// contain digits and letters (either lowercase or uppercase)
public static string GetRandomPassword(int length)
{
Random rand = new Random();
System.Text.StringBuilder password = new System.Text.StringBuilder(length);
for (int i = 1; i <= length; i++) { int charIndex; // allow only digits and letters do { charIndex = rand.Next(48, 123); } while (!((charIndex >= 48 &&amp; charIndex <= 57) (charIndex >= 65 && charIndex <= 90) (charIndex >= 97 && charIndex <= 122))); // add the random char to the password being built password.Append(Convert.ToChar(charIndex)); } return password.ToString(); }
Step5: Write Code for Generating Image. This code will create RegistrationImg.gif in your application folder.
//Generate Image
private void GenerateImage(string strRegistrationStr)
{
Bitmap objBitmap = new Bitmap(150, 30);
Graphics objGraphics = Graphics.FromImage(objBitmap);
//Fore Color and Background of Image
SolidBrush objForeColor = new SolidBrush(Color.White);
SolidBrush objBackColor = new SolidBrush(Color.Black);
objGraphics.FillRectangle(objBackColor, 0, 0, 150, 30);
//Font settings for Image
Font objFont = new Font("Arial", 15);
//Display from 5point from x-axis and 5point from y-axis
Point objPoint = new Point(5, 5);
//Drawing Registration String
objGraphics.DrawString(strRegistrationStr, objFont, objForeColor, objPoint);
//Saving Registration String as Image
//If you dont want image to save
//objBitmap.Save(Response.OutputStream, ImageFormat.Gif);
//otherwise use this
objBitmap.Save(Server.MapPath("RegistrationImg.gif"), ImageFormat.Gif);
//Release object
if(objBitmap != null)
objBitmap.Dispose();
if (objGraphics != null)
objGraphics.Dispose();
}
Step6: Finally code for calling the created functionality.
protected void btnShowRegistrationImg_Click(object sender, EventArgs e)
{
GenerateImage(GetRandomPassword(10));
ImgRegistrationStr.ImageUrl = "RegistrationImg.gif";
}