Exploring LLBL Gen. Pro.
Want to know what is LLBL Gen. Pro. ??? than click here.
Using LLBL Gen. Pro. tool is divided into Three parts
Part 1: Discuss All about Generating Files from LLBL Tool.
Part 2: Execute StoredProcedure in Database.
Part 3: Discussion about using files generated from LLBL Tool.
Part1
Step1: Enter DB Server., user-id and password.
Step2: Select Table.
Step3: Select Language option and namespace. Select DBNull value support and property option rather than web.config file option.
Step4: Select the field names
Step5: Start Generation : It will generate three files clsCustomer.cs, clsDBInteractionBase.cs and Northwind_LLBL_StoredProcedures.txt
clsCustomer.cs - It consist of functions for SelectOne, SelectAll, Update, Delete, Insert, Update.
clsDBInteractionBase.cs - It is a abstract class which needs to be included once for all the tables, it consist of functions such as BeginTransaction, CommitTransaction, RollbackTransaction, and so on which are require for DBInteraction.
Step1: Open QueryAnalyzer, and select the database. (here choose Northwind).
Step2: Copy all the content from "Northwind_LLBL_StoredProcedures" file and execute it in query analyser.
Part3
Step1: Create New Web Project (you can use it with window project, but for this example i am going to explain with Web Project).
Step2: Add New Class Library project. (you can make individual class library project outside main project and add its .dll file as reference)
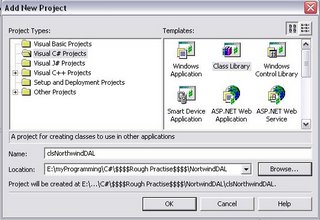
In InitClass() method, change m_ScoMainConnection.ConnectionString = ConfigurationSettings.AppSettings["connStr"].ToString();
where "connStr" is a key for connection string define in web.config file.
This change will help you to use connection string from web.config file.
Also, if required, In clsCustomer.cs file replace Int32 with SqlInt32.
Step5: Add the reference of class project within your web project to use its functionality. Right click on web project and click on add reference and add choose project tab., add the Northwind Class.
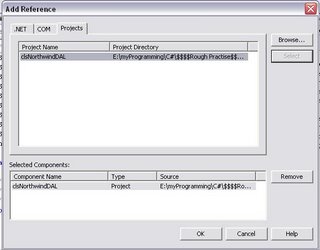
Step7: Switch to Code view and add namespace of generated file.
eg: using NorthwindLLBL;
Step8: Add code to display all the data of customer in datagrid.
eg:
if(!Page.IsPostBack)
{
clsCustomers objCust = new clsCustomers();
DataGrid1.DataSource = objCust.SelectAll();
DataGrid1.DataBind();
}